Sunday, December 24, 2006
Maximum URL length in HTTP request.
But now, in HTTP 1.1 length of URL is 2,083 characters.
Limiting only to GET. For POST a length is not limited.
Original
Monday, December 11, 2006
Custom object and ToString()
That is one solution :
public int Id
{
get { return id; }
set { id = value; }
}
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
private DateTime birthday ;
public DateTime Birthday
{
get { return birthday ; }
set { birthday = value; }
}
//Constructor
public Customer()
{
this.id = 5;
this.name = "John Smith";
this.birthday = new DateTime(1945, 10, 12);
Console.WriteLine(this.ToString());
}
To continue press "read more"....
public override string ToString()
{
StringBuilder builder = new StringBuilder();
Type type = this.GetType();
PropertyInfo[] propertiesInfo =
type.GetProperties();
foreach (PropertyInfo property in propertiesInfo)
{
builder.AppendFormat("{0} = {1} \n",
property.Name,
property.GetValue(this, null));
}
return builder.ToString();
}
Output:
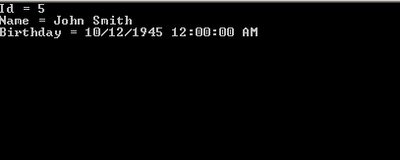
Thursday, November 30, 2006
Writing log message to file via Message Queue
using System;
using System.Configuration;
using System.Messaging;
using System.IO;
namespace Test
{
public class QSend
{
private System.Messaging.MessageQueue mq;
private string exQ;
public QSend()
{
try
{
mq = new System.Messaging.MessageQueue(
@".\Private$\MyQ");
LogItems inLogItems = new LogItems();
inLogItems.FileName = @"d:\mgslog\Log.txt";
inLogItems.Message = " my message";
mq.Send(inLogItems);
}
catch(MessageQueueException ex)
{
exQ = ex.Message;
}
}
}
public class QRecieve
{
private System.Messaging.MessageQueue mq;
private string exQ;
public QRecieve()
{
try
{
mq = new System.Messaging.MessageQueue (@".\Private$\MyQ");
mq.Formatter =
new System.Messaging.XmlMessageFormatter (
new Type[] {typeof (LogItems)}) ;
System.Messaging.Message msg = mq.Receive();
LogItems outLogItems = (LogItems) msg.Body;
using( StreamWriter sw =
new StreamWriter(outLogItems.FileName,true))
{
sw.WriteLine(DateTime.Now.ToString()
+ outLogItems.Message);
sw.Flush();
}
}
catch(MessageQueueException e)
{
exQ = e.Message;
}
}
}
public class LogItems
{
private string _fileName;
private string _message;
public string FileName
{
get {return _fileName;}
set {_fileName = value;}
}
public string Message
{
get {return _message;}
set {_message = value;}
}
}
}
Tuesday, November 21, 2006
Convert any possible type to T in .Net 2005
{
T convertedValue = defaultValue;
try
{
if (o != null)
{
convertedValue = (T)Convert.ChangeType(o, typeof(T));
}
else
throw;
}
catch
{
if (isThrowException)
{
throw;
}
}
return convertedValue;
}
How to use:
Let us suppose you have HttpRequest with some parameters:
ID , Name and Flag
Example 1. Simple using
private void Examle()
{
int id =
TryConvert<int>(Request["id"], -1,
true);
string name =
TryConvert<string>(Request["name"], string.Empty,
true);
bool flag =
TryConvert<bool>(Request["Flag"], default(bool),
true);
}
Example 2. Using in enum
public enum Week
{
Monday,
Tuesday,
Wednesday,
Thursday,
Friday,
Saturday,
Sunday
}
private void Examle2(Week w)
{
Week day = TryConvert<Week>(w, Week.Sunday, true);
}
Thursday, October 26, 2006
NMock
Home page
Comparison of logging services.
Microsoft Enterprise Library
Log4net
Syslog
The scenario of the test:
1. Reading of a line from a DB.
2. Record of a line in a DB.
Here results on 500 inquiries:
Log4Net | Enerprise Library | Syslog | |
Concurrency Level | 25 | 25 | 25 |
Time taken for tests | 1.617758 seconds | 1.794552 seconds | 1.270281 seconds |
Complete requests | 500 | 500 | 500 |
Failed requests | 0 | 0 | 0 |
Write errors | 0 | 0 | 0 |
Total transferred | 566968 bytes | 701000 bytes | 695000 bytes |
Requests per second | 309.07 [#/sec] (mean) | 278.62 [#/sec] (mean) | 393.61 [#/sec] (mean) |
Time per request | 80.888 [ms] (mean) | 89.728 [ms] (mean) | 63.514 [ms] (mean) |
Time per request(mean, across all concurrent requests) | 3.236 [ms] | 3.589 [ms] | 2.541 [ms] |
Transfer rate | 341.83 Kbytes/sec] | 381.15 [Kbytes/sec] | 533.74 [Kbytes/sec] |
Wednesday, September 20, 2006
HttpHandler in WebSite VS 2005
This example intended to case, when classes located in App_Code.
HttpHandler:
public class TestHandler : IHttpHandler
{
public TestHandler() {}
public void ToDo()
{
//....
}
public bool IsReusable
{
get { return true; }
}
void IHttpHandler.ProcessRequest(
HttpContext context)
{
ToDo();
}
}
Web.Config:
<httpHandlers>
<add path="test.test" verb="*"
type="TestHandler, App_Code" />
</httpHandlers>
Thursday, July 27, 2006
Add an uninstall and him shortcut to .NET deployment project in VS2005
2. Add in Main()
{
string[] arguments = Environment.GetCommandLineArgs();
foreach(string argument in arguments)
{
string[] parameters = argument.Split('=');
if (parameters[0].ToLower() == "/u")
{
string productCode = parameters[1];
string path = Environment.GetFolderPath(Environment.SpecialFolder.System);
Process proc = new Process();
proc.StartInfo.FileName = string.Concat(path,"\\msiexec.exe");
proc.StartInfo.Arguments = string.Concat(" /i ", productCode);
proc.Start();
}
}
3. Create new setup project
4. Add UninstallApp.exe to "Application Folder" in 'File System' part
5. In "User's Program menu" create shortcut to UninstallApp.exe and in properties of this shortcut in parameter 'arguments' insert value "/u=[ProductCode]".
6.Rebuild deployment project.
Good luck ;)
Friday, June 16, 2006
ILoveJackDaniels.com
Sunday, June 04, 2006
Buttons
http://www.buttonator.com/
Clean AJAX
Clean AJAX - is an open source engine for AJAX which, is technology of creation asynchronous HTTP requests. Clean AJAX is open source and released under a GNU General Public License, download is available from souceforge.
For more information : Click here
Wednesday, May 17, 2006
Event Log Explorer
Download(1.0 MB)
Wednesday, May 10, 2006
HTMLCrypt 2.60
Download
Thursday, May 04, 2006
SqlDependency in VS.NET 2005
SqlCommand command = new SqlCommand("Select * from Table");
// Clear any existing notifications
command.Notification = null;
.
.
.
// Create the dependency for this command
SqlDependency dependency = new SqlDependency(commmand);
// Add the event handler
dependency.OnChange += new OnChangeEventHandler(OnChange);
voidOnChange(object sender, SqlNotificationEventArgs e)
{
SqlDependency dependency = sender as SqlDependency;
dependency.OnChange += OnChange;
//Handler for Object
OnAnyEvent();
}
Monday, May 01, 2006
Storing Code Snippets in VS.NET
Add a code snippet to a tab of the Toolbox as follows:
- 1.Select the code snippet in the code file
- 2.Copy the text.
- 3.Click the desired tab on the Toolbox.
- 4.Right-click the area below the tab.
- 5.Select "Paste" from the context menu.
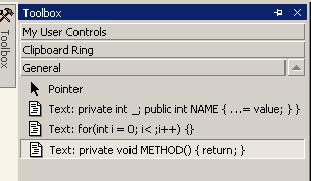
Sunday, April 30, 2006
Split in SQL
Split list of numbers ('1, 2, 3, 4, 67, 87') to table.
Input: : List of numbers (0;1;5;45;...)
Ouput:
0 | 1 | 5 | 45 | ... |
A using: SELECT * FROM Split('0;1;5;45;',';')
(
@list varchar(8000), -- List of integer with any delemiter
@delimiter CHAR(1) -- Delimetr
)
RETURNS
@list_int TABLE (item int) --list of integer
AS
BEGIN
DECLARE @position int, @itemvarchar(20)
WHILE @list <> ''
BEGIN
SET @position = CHARINDEX(@delimiter, @list)
IF @position = 0
SET @position = LEN(@list) + 1
SET @item=LEFT(@list, @position - 1)
INSERT INTO @list_int VALUES(CAST(@itemAS int))
SET @list = STUFF(@list, 1, @position, '')
END
RETURN
END
Use of conditional attributes in .NET
[Conditional ("FINAL")]
public static void Hello() { Console.WriteLine("Thanks for registration!"); Console.ReadLine(); }
Add to project properties -> Build -> Conditional compilation constants :
DEBUG;TRACE; TRIAL "COLOR: #ff0000">or DEBUG;TRACE; FINAL Compile and Run.
Tuesday, April 25, 2006
Google advanced operators
Target/Task | links | Uses operators |
What Google site writes about blog? | site:google.com inanchor:blog | site: inanchor: |
Find car BMW in UK | Results from all word: BMW Result from UK and other link: BMW UK Results from UK only: inurl:co.uk intitle:BMW | Inurl: |
Find info about bugs | Any bugs: Bugs Any bugs except of computer bugs: Bugs -computer | Exclusion |
Understand the DOT.NET dataset class structure | dataset | Google images |
What Blog is? | Define: blog | define |
Find info about mobile/cellular phones | Intitle: phones intitle:~mobile | Synonyms(~) |
Find PowerPoint presentation about cars/auto | ~car filetype:ppt | Synonyms(~), filetype |
Find any installation guide in flash | "installation guide" filetype:swf | Exact match(""), filetype |
Find middle Vladimir Putin name | "Vladimir * Putin" | Asteric(*) |
Effect of incomming links | Click here (Adobe, XE, Macromedia) |
List of operators:
Intitle,allintitle | Inurl, allinurl | Filetype | Allintext | Site |
Link | Inanchor | Daterange | Cache | Info |
Related | Phonebook | Rphonebook | Bphonebook | Author |
Group | define | Msgid | Insubject | Stocks |
Sunday, April 23, 2006
Exact measurement of performance in Dot.Net
Measures much more precisely than Environment.TickCount. Environment.TickCount has accuracy 10 milisecond,
that for measurement of short sites does not approach.
Use QueryPerformanceCounter allows to provide very high accuracy.
To use so:
//Define variable PerfMeter timer = new PerfMeter(); timer.Start(); // A code for test....... //Print result to console Console.WriteLine("Execution time in seconds: {0:### ### ##0.0000}" timer.End()); //You can to use one variable several times |
Class code:
using System; using System.Runtime.InteropServices; namespace Utils { /*This structure allows to count up speed of performance of a code one of the most exact of ways. Actually calculations are made in steps of the processor, and then translated in miliseconds (the decimal part is shares of second). */ public struct PerfMeter { Int64 _start; /// Start timer public void Start() { _start = 0; QueryPerformanceCounter(ref _start); } /// Finished calculation time executions and returns time in seconds. /*Return: Time in seconds spent for performance of a site of a code. The decimal part reflects shares of second */ public float End() { Int64 end = 0; QueryPerformanceCounter(ref end); Int64 frequency = 0; QueryPerformanceFrequency(ref Frequency); return (((float)(end - _start) /(float)freq)); } [DllImport("Kernel32.dll")] static extern bool QueryPerformanceCounter(ref Int64 performanceCount); [DllImport("Kernel32.dll")] static extern bool QueryPerformanceFrequency(ref Int64 frequency); } } |
BIOS error signals
During inclusion of a computer the sound signal is always audible, but sometimes it is more, than a lonely signal. You what is it? Ask, and the answer will be not pleasant - there was a problem with hardware. This signal publishes BIOS, and values of signals are resulted in tables below:
AMI
Signals | Problem |
- * | Power Supply is out of order |
2 short | Clock memory error |
3 short | Error in first 64kb of memory |
4 short | System timer error |
5 short | CPU is out of order |
6 short | Keyboard controller is out of order |
7 short | Motherboard is out of order |
8 short | VGA card is out of order |
9 short | Test BIOS error |
10 short | Can not write to CMOS |
11 short | Motherboard cache memory is out of order |
1 long + 2 short | VGA card is out of order |
1 long + 3 short | Same |
1 long + 8 short | The monitor is not connected. |
AWARD
Signals | Problem |
2 short | little errors** |
3 short | Keyboard conroller error |
1 long +1 short | Memory error |
1 long + 2 short | VGA card is out of order |
1 long + 3 short | Keyboard initialization error |
1 long + 9 short | Read error from ROM |
short, recurrent | Power Suplly is out of order |
long, recurrent | Memory problem |
continual | Power Suplly is out of order |
* - a absence of any signal.
** - Problem in CMOS Setup or motherboard.